PDF Tool APIサンプル集:ページサイズに合わせて折り返す文字列(新規のページを作成)
PDFの出力先となるファイルパスを指定して 新規のPDFのページを用意し
ページサイズに合わせて折り返す文字列を書きこんで出力するコンソールアプリケーションです。
概要
コマンドラインでの実行例
sample.exe c:\sav\out.pdf
ダウンロード
出力結果イメージ
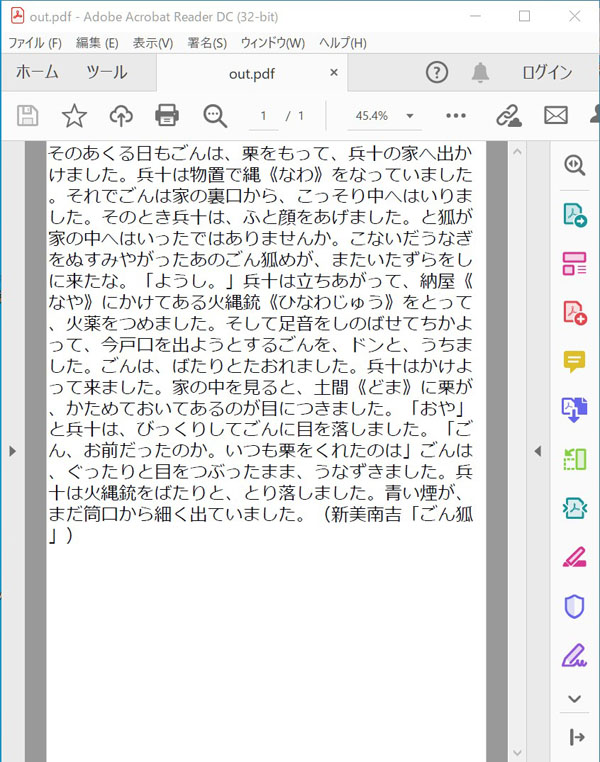
サンプルコード
/* Antenna House PDF Tool API 7.0 C# Interface sample program 概要:ページサイズに合わせて折り返す文字列(新規のページを作成) Copyright 2021 Antenna House,Inc. */ using System; using PdfTkNet; namespace sample04cs { class Program { static void Main(string[] args) { Console.WriteLine("PDF Tool API V7.0 C# サンプル"); // 出力ファイル名の初期値を設定 string outFilePath = @"C:\sav\out.pdf"; // 出力ファイル名 if (args.Length > 0) { outFilePath = args[0]; } try { using (PtlParamOutput output = new PtlParamOutput(outFilePath)) using (PtlPDFDocument doc = new PtlPDFDocument()) using (var option = new PtlOption()) { option.setUnit(PtlOption.UNIT.UNIT_PT); string fontName = "メイリオ"; float fontSize = 24.0f; using (PtlParamFont fontNormal = new PtlParamFont(fontName, fontSize, false, false, true)) using (PtlColorDeviceRGB colorBlack = new PtlColorDeviceRGB(0.0f, 0.0f, 0.0f)) using (PtlPages pages = doc.getPages()) using (PtlPage newpage = new PtlPage()) using (PtlSize pageSize = newpage.getSize()) { pages.append(newpage, PtlPages.INSERT_OPTION.OPTION_NONE); // 新規ページの追加 using (PtlContent content = newpage.getContent()) using (PtlRect rect = new PtlRect(0, 0, pageSize.getWidth(), pageSize.getHeight())) using (PtlTextBox textBox = content.drawTextBox(rect, PtlContent.ALIGN.ALIGN_TOP_LEFT, pageSize.getWidth(), pageSize.getHeight())) using (PtlParamWriteStringTextBox paramWriteString = new PtlParamWriteStringTextBox()) { paramWriteString.setFont(fontNormal); paramWriteString.setTextColor(colorBlack); string text1 = "そのあくる日もごんは、栗をもって、兵十の家へ出かけました。兵十は物置で縄《なわ》をなっていました。それでごんは家の裏口から、こっそり中へはいりました。そのとき兵十は、ふと顔をあげました。と狐が家の中へはいったではありませんか。こないだうなぎをぬすみやがったあのごん狐めが、またいたずらをしに来たな。「ようし。」兵十は立ちあがって、納屋《なや》にかけてある火縄銃《ひなわじゅう》をとって、火薬をつめました。そして足音をしのばせてちかよって、今戸口を出ようとするごんを、ドンと、うちました。ごんは、ばたりとたおれました。兵十はかけよって来ました。家の中を見ると、土間《どま》に栗が、かためておいてあるのが目につきました。「おや」と兵十は、びっくりしてごんに目を落しました。「ごん、お前だったのか。いつも栗をくれたのは」ごんは、ぐったりと目をつぶったまま、うなずきました。兵十は火縄銃をばたりと、とり落しました。青い煙が、まだ筒口から細く出ていました。(新美南吉「ごん狐」)"; textBox.writeStringNL(text1, paramWriteString); // 文字列を出力して改行 textBox.terminate(); } doc.save(output); Console.WriteLine("-- 完了 --"); } } } catch (PtlException pex) { Console.WriteLine(pex.getErrorCode() + " : " + pex.getErrorMessageJP()); pex.Dispose(); } catch (Exception ex) { Console.WriteLine(ex.Message); } } } }